2 Minutes to Learn React 16's componentDidCatch Lifecycle Method
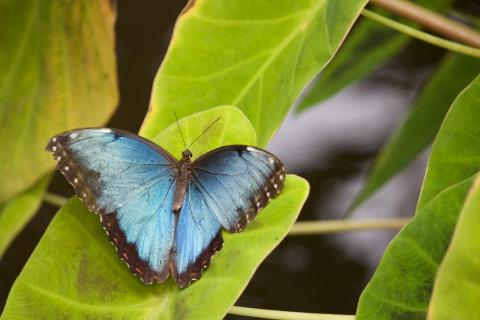
What are Error Boundaries?
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. Error boundaries catch errors during rendering, in lifecycle methods, and in constructors of the whole tree below them. -Dan Abramov
You can create an error boundary class component by defining a new lifecycle method componentDidCatch(error, errorInfo)
.
componentDidCatch() Lifecycle Method
componentDidCatch(error, errorInfo) {}
The first method parameter is the actual error thrown. The second parameter is an object with a componentStack
property containing the component stack trace information. The componentDidCatch()
lifecycle method works similar to JavaScript's catch {}
but for components. An Error Boundary cannot catch an error within itself. The error will propagate to the closest Error Boundary above it. Sound familiar?
hint: JavaScript catch {}
block.
Why do I need componentDidCatch()?
Prior to React 16 Error Boundaries, errors inside components would cause unrecoverable cryptic errors. There was not a great way to handle these errors within the components.
React 16 Error Boundaries to the rescue!
Benefits:
- Declarative vs Imperative. One of the beauties of React is its Declarative code style. A component itself declares what should be rendered. Error Boundaries follow the Declarative nature of React. You no longer need to resort to imperative code solutions such as
try/catch
orif/else
for conditional rendering of a UI based on errors. - Expected Behavior. Error Boundaries will propagate an error to the closest Error Boundary regardless how deep in the tree the error occurs.
- Reusability. You can write one Error Boundary Component and reuse it throughout your app.
- Component All The Things
Issues:
¯_(ツ)_/¯
How do I use Error Boundaries?
I've created a CodePen to demo the componentDidCatch()
lifecycle method. I'd highly recommend forking the pen and adding more Error Boundaries and components to see them in action.
https://codepen.io/sgroff04/pen/dVbgJy/
When should I use Error Boundaries?
In practice, most of the time you'll want to declare an error boundary component once and use it throughout your application. -Dan Abramov
You can wrap top level route components, you might also wrap components that might contain obscure code. It's up to you how to best handle protecting against application crashes.
Once React 16 is released more standards and best practices with Error Boundaries will appear. Error Boundaries will encourage JavaScript error reporting services that can be triggered within the componentDidCatch()
lifecycle method in Production environments.
For more details check out Error Handling in React 16 by Dan Abramov.
Want to talk about how we can work together?
Ryan can help
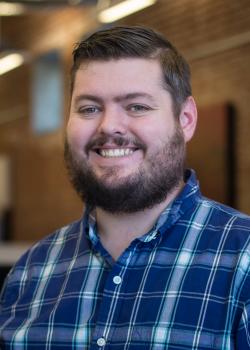